Limited Time Offers (LTO)
Preparation
Remember, you need to configure and initialize the Magify Service to work with purchases and subscriptions.
Campaigns are available only with advanced Magify SDK integration.
You should also read up on campaigns in general, because LTO is a subset of campaigns.
Main idea
Often apps need to implement some sort of limited time offer. This can be temporary promotions, sales, limited time to purchase content and other similar examples. If you are already familiar with the concept of campaigns, it should be clear to you that if you add a time limit to campaigns, you will get just such offers.
Technical LTO's are just an extension of the campaigns functionality. However, they also bring a lot of functionality with them, which will be described in more detail below.
Implementation
The main difference between LTO and common campaigns is the way they are visually represented and processed.
Launching an LTO is pretty much the same as launching a regular company. At the required location you have to call MagifyService.RequestCampaignAsync method and if all conditions are matched and the LTO can be launched, it will be launched. In this case, you will be returned the result of the campaign request, but on top of that, the LTO will start its lifecycle inside the SDK.
By default, the SDK provides a base class LimitedTimeOfferBase for working with offers. It is an inheritor of MonoBehaviour
. And it is a default way for displaying LTO. Since it is an abstract class you must create a class that inherits from it and implement abstract members. And also non-abastract LimitedTimeOffer
class for the simplest variant of LTO processing.
Using default solution
The easiest way to implement LTO would be to attach LimitedTimeOffer
to some game object on your scene. In this example, it's just a static object that is predefined in your application.
Then, while the application is running, you call the MagifyService.RequestCampaignAsync method, and if the LTO campaign was launched with the same spot as the one configured in the 'Offer Spot' field, then this object will be notified by SDK and the display of the launched offer will start.
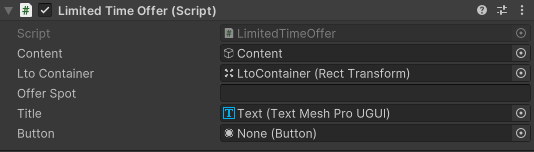
Using LTO via scripts
Another use case for LTOs would be to create and customize them with code.
First, let's create inheritor of the LimitedTimeOffer
class and add a method to set the OfferSpot
from outside:
public class ExampleLto : LimitedTimeOffer
{
public void SetSpot(string spot)
{
OfferSpot = spot;
}
}
Now we can create some class (LtoContainer
) that will contain all the LTO objects on the current application screen. We will keep track of changes in the list of active offers and create or destroy objects depending on the added and deleted items:
public class LtoContainer : MonoBehaviour
{
[SerializeField]
private ExampleLto _ltoPrefab;
[SerializeField]
private Transform _parent;
protected async void Start()
{
var magify = await MagifyService.WaitForInitialized();
magify.Offers.ActiveOffers.ObserveAdd()
.TakeUntilDestroy(this)
.Subscribe(ltoInfo =>
{
_ltoPrefab.gameObject.SetActive(false); // because LimitedTimeOfferBase is trying to initialize on Awake(), but we need to set the spot before
var instance = Instantiate(_ltoPrefab, _parent);
_ltoObjects.TryAdd(ltoInfo.Value.Spot, instance);
instance.SetSpot(ltoInfo.Value.Spot);
instance.gameObject.SetActive(true); // here offer will be initialized with given spot
_ltoPrefab.gameObject.SetActive(true); // if necessary
});
magify.Offers.ActiveOffers.ObserveRemove()
.TakeUntilDestroy(this)
.Subscribe(ltoInfo =>
{
if (_ltoObjects.Remove(ltoInfo.Value.Spot, out var instance))
Destroy(instance.gameObject);
});
}
}
In this option, you don't need to predefine the position of all the offers, you just need to properly position them within the container as they are added and removed. This adds flexibility to your interface.